Big Picture First
Ok, as described in the concept post, this box is going to have a series of software packages installed to enable it to do cool stuff all damn day. I’m a fan of open source and so all of these are coming from GitHub. If you’re not already familiar with GitHub, you’re missing out on some awesome projects.
- Birdnet-Pi
- This is the package that listens during the day (and at night too) for birdsong. I’ve found the Nachtzuster version to be the more updated and functional one, especially if you’re running on newer hardware.
- Allsky Camera
- I like the AllskyTeam software package available at GitHub. It’s mostly focused on taking pretty pictures of the sky which is where I’m starting. I might migrate to another one later on if I want to start triangulating meteors…
- Adafruit INA3221 Python Libraries
- This package is to enable us to monitor the power levels in and out of the solar panel, batteries, and USB-C output to the RPi. As noted in the hardware post, I went with Adafruit implementation because has three buses, monitors voltage and current, and can do both high or low side monitoring. It also helps that they provide these awesome software packages and instructions on how to use them.
Implementation (WORK IN PROGRESS, USE AT YOUR OWN RISK)
Hook up hardware, yes all of it.
Flash drive with latest OS-lite using whatever tool you prefer. If this is your first time with a Raspberry Pi I recommend following their instructions to make it easier.
If you’re directly connected to your Raspberry Pi you can setup the rest of the system once it boots. If you’re doing this via SSH, I probably don’t need to tell you but make sure to setup your wifi settings and users in the configuration prior to writing to the SD card.
Birdnet Installation
Install Nachtzuster Birdnet as directed.
Keep installing, it will reboot when installation is complete.
Check out your Birdnet installation to make sure it’s generally working. You should see some lines on the audio plots.
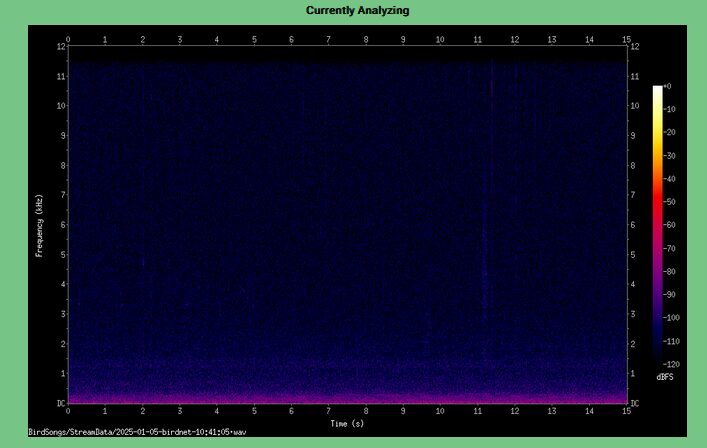
If Birdnet isn’t working, do not proceed. Birdnet will not install itself nicely on top of other software packages. You have to get this working first!
Allsky Installation
Assuming Birdnet is working, move on to Allsky installation with some modifications:
Git was installed with Birdnet so you don’t need to install it per the Allsky instructions, just jump into installing the Allsky software.
cd
git clone --recursive https://github.com/AllskyTeam/allsky.git
cd allsky
./install.sh
Answer questions (fresh install, camera connected, ram disk)
Error Code recorded:
Job for lighttpd.service failed because the control process exited with error code.
See "systemctl status lighttpd.service" and "journalctl -xeu lighttpd.service" for details.
Eventually it will ask for some location data, input it.
Couple more notices
Allow system to reboot after install
Custom Configurations
Pull up a command line on your RPi (SSH or local), username is admin
, password secret
Configure Allsky Settings
Cd /etc/lighttpd/
Sudo vi lighttpd.conf
Update server.port=80
to server.port=81
This moves the Allsky server to port 81 when you’re using a web browser to access the system.
Birdnet will automatically continue running on port 80
Setup CircuitPython
Following these steps to install blinka first https://learn.adafruit.com/circuitpython-on-raspberrypi-linux/installing-circuitpython-on-raspberry-pi
The instructions assume you’re installing from a fresh OS with no extra software but because we’ve already installed two modern software packages we’ve got some stuff already setup. Namely PIP, Setuptools, and the python virtual environment (venv).
python3 -m venv env --system-site-packages
source env/bin/activate
install via automated installer
Reboot
Reconnect to the RPi
This step may not be needed, I need to investigate further. Won’t hurt though if you do run it (I did).
python3 -m venv env –system-site-package
Here’s the important steps though. This sets up your project and environment for Blinka.
mkdir project-name && cd project-name
python3 -m venv .env
source .env/bin/activate
pip3 install Adafruit-Blinka
sudo nano blinkatest.py
import board
import digitalio
import busio
print("Hello, blinka!")
# Try to create a Digital input
pin = digitalio.DigitalInOut(board.D4)
print("Digital IO ok!")
# Try to create an I2C device
i2c = busio.I2C(board.SCL, board.SDA)
print("I2C ok!")
# Try to create an SPI device
spi = busio.SPI(board.SCLK, board.MOSI, board.MISO)
print("SPI ok!")
print("done!")
Exit out of nano (or whatever text editor you’re using). Then run it with:
python3 blinkatest.py
You should get this:
(.env) adminaccount@NaturePi:~/power_monitor$ python3 blinkatest.pyHello, blinka!
Digital IO ok!
I2C ok!
SPI ok!
done!
That’s success right there!
Now we’ll install the actual code we need for the INA3221 board. Install the library required with this command:
pip3 install adafruit-circuitpython-ina3221
Create a new file called powermonitor.py and open it in a text editor:
sudo nano powermonitor.py
Here’s the Adafruit code, again why make life hard when you can just use it?
# SPDX-FileCopyrightText: Copyright (c) 2024 Liz Clark for Adafruit Industries
#
# SPDX-License-Identifier: MIT
import time
import board
from adafruit_ina3221 import INA3221
i2c = board.I2C()
ina = INA3221(i2c)
while True:
for i in range(3):
bus_voltage = ina[i].bus_voltage
shunt_voltage = ina[i].shunt_voltage
current = ina[i].current_amps * 1000
print(f"Channel {i + 1}:")
print(f" Bus Voltage: {bus_voltage:.6f} V")
print(f" Shunt Voltage: {shunt_voltage:.6f} V")
print(f" Current: {current:.6f} mA")
print("-" * 30)
time.sleep(2)
Should see power data, CTRL-x to break.
Leave a Reply